Looking to utilize Steam Enhanced Rich Presence with Unreal Engine 5? Check out the tutorial below to get started.
I’m working on an online multiplayer RPG called Moongate, and wanted to take advantage of Steamwork’s Rich Presence to communicate certain aspects of the game via the friends list.
The rich presence attributes aren’t blueprint accessible by default, so we’re going to create a new Game Instance class in C++, expose those variables to blueprint, and create a localization file in Steamworks to set up rich presence in Unreal Engine 5.
Pre-requisites:
Unreal Engine 5.4
Advanced Steam Sessions
Visual Studio
Steamworks (and Steamworks associated Developer ID)
First things first: create a new C++ class in Unreal Engine, and set the base class as Game Instance. This is initialized throughout the gameplay state, meaning we can start using rich presence throughout the entire game.
If you’re new to C++, you’ll need a header, which is essentially the definitions of the program you’re creating, and the C++, which takes those definitions and turns them into logic.
Header
GameInstanceSteamPresence.h:
#pragma once
#include "CoreMinimal.h"
#include "Engine/GameInstance.h"
#include "GameInstanceSteamPresence.generated.h"
UCLASS()
class YOURPROJECTNAME_API UGameInstanceSteamPresence : public UGameInstance
{
GENERATED_BODY()
public:
UFUNCTION(BlueprintCallable, Category = "GameInstance")
void SetSteamRichPresence(const FString& Key, const FString& Value);
UFUNCTION(BlueprintCallable, Category = "GameInstance")
void ClearSteamRichPresence();
};
CPP
GameInstanceSteamPresence.cpp:
#include "GameInstanceSteamPresence.h"
#include "ThirdParty/Steamworks/Steamv157/sdk/public/steam/steam_api.h"
void UGameInstanceSteamPresence::SetSteamRichPresence(const FString& Key, const FString& Value)
{
if (SteamAPI_Init() && SteamFriends() != nullptr)
{
// Attempt to set Steam Rich Presence
SteamFriends()->SetRichPresence(TCHAR_TO_UTF8(*Key), TCHAR_TO_UTF8(*Value));
UE_LOG(LogTemp, Log, TEXT("Attempting to set Steam Rich Presence for key: %s, value: %s"), *Key, *Value);
}
else
{
UE_LOG(LogTemp, Warning, TEXT("Steam is not running or the SteamFriends interface is unavailable"));
}
}
void UGameInstanceSteamPresence::ClearSteamRichPresence()
{
if (SteamAPI_Init() && SteamFriends() != nullptr)
{
// Attempt to clear Steam Rich Presence
SteamFriends()->ClearRichPresence();
UE_LOG(LogTemp, Log, TEXT("Attempting to clear Steam Rich Presence"));
}
else
{
UE_LOG(LogTemp, Warning, TEXT("Steam is not running or the SteamFriends interface is unavailable"));
}
}
There are probably better ways of accomplishing this, but probably few that are as direct or immediately functional.
Compile, and re-open your project. If you have a custom game instance already, re-parent it to our new game instance class, GameInstanceSteamPresence. Otherwise, assign GameInstanceSteamPresence as your base Game Instance.
Create as many statuses as needed. Take a look at DOTA 2 or Path of Exile’s
implementation for inspiration.
I plan on creating a bunch of statuses to reflect various kinds of activities and adventures, including things like health or whether or not your character is still alive, but we’re not there yet. Instead, let’s just choose one of four and change it every couple of minutes.
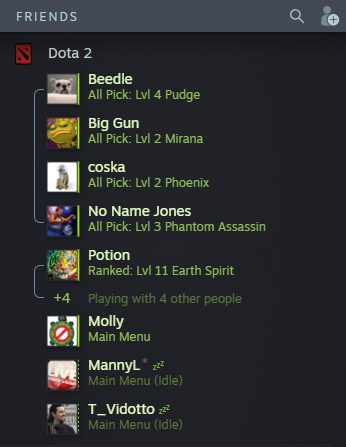
Connect this to your Event Begin Play, and this event will trigger at the start of the game, and re-trigger every 6 minutes, choosing a number between 1 and 4.
Blueprint Setup
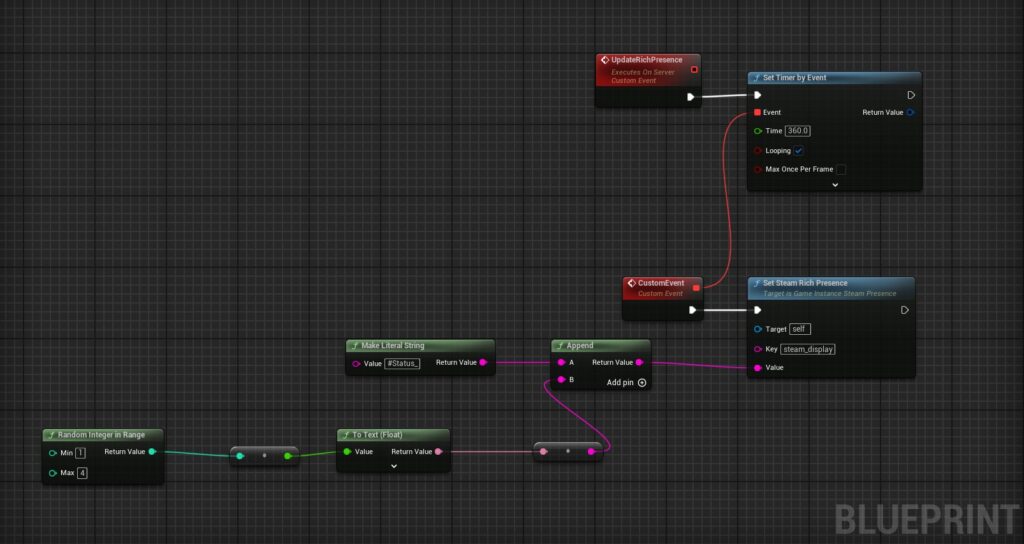
Next, we create a simple json file, which we will upload as localization to Steamworks. Here’s an example of how Steam is anticipating the json to look:
JSON
"lang"
{
"Language" "english"
"Tokens"
{
"#Status_01" "Embarking on a quest"
"#Status_02" "Waiting for a Quest"
"#Status_03" "Exploring beyond the Ghost Wood"
"#Status_04" "Destroying an Ancient Lich's Phylacteries"
}
}
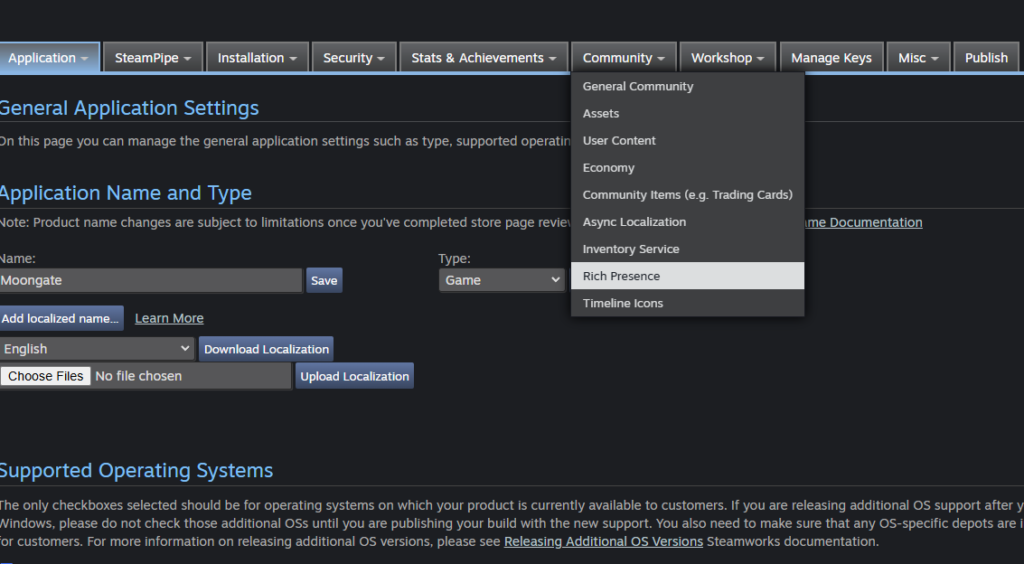
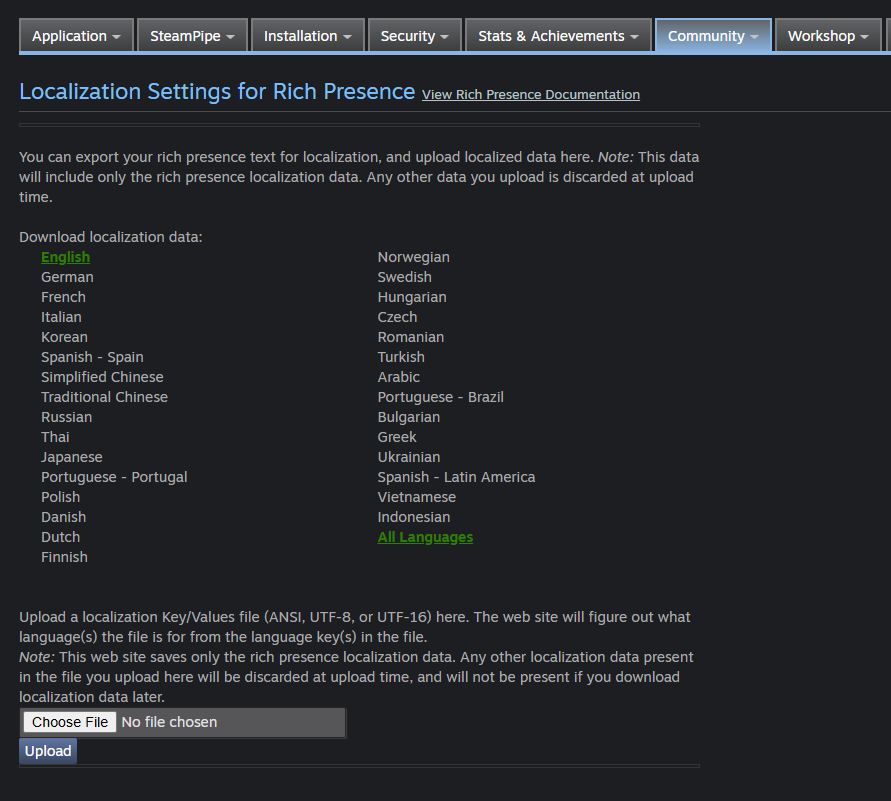
And that’s it.
Your Game Instance sends updates to Steam, telling it which status to use as your rich presence text, which then pushes that to your steam friends status. Steamworks documentation provides a more comprehensive guide on how to dynamically tie game information into the rich presence status, reade more here: https://partner.steamgames.com/doc/features/enhancedrichpresence